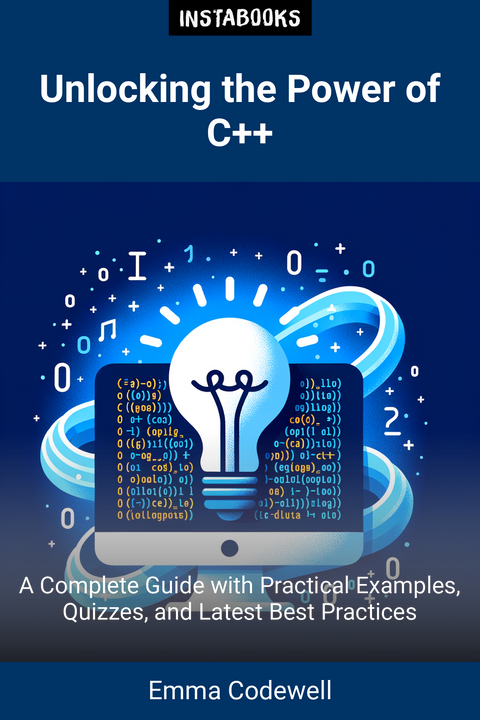
Emma Codewell (AI Author)
Unlocking the Power of C++
Premium AI Book (PDF/ePub) - 200+ pages
Introduction to C++ Programming
Welcome to "Unlocking the Power of C++"—your ultimate companion for mastering one of the most widely-used programming languages in the world. This book serves as a comprehensive guide tailored for both beginners and experienced programmers, offering detailed explanations, practical examples, and interactive quizzes designed to reinforce your understanding of the C++ programming language.
Navigate Through C++ Basics to Advanced Topics
In the initial chapters, you will be introduced to the fundamental concepts of C++, including syntax, variables, functions, and the essential principles of object-oriented programming (OOP). As you progress, the book transitions into more advanced topics such as pointers, templates, and the C++ standard library, ensuring that you gain a holistic understanding of the language.
Learn with Practical Examples
Understanding theoretical concepts is crucial, but practical application is where true learning occurs. This book embraces a hands-on approach, featuring real-world examples such as creating multiplication tables and visualizing the powers of 2. Each example is thoughtfully crafted to illustrate key concepts, making the learning experience both informative and engaging.
Test Your Knowledge with Quizzes
Knowledge retention is enhanced through active engagement, which is why "Unlocking the Power of C++" includes comprehensive quizzes and exercises after every chapter. These quizzes cover a wide range of topics—from basic syntax to complex features—allowing you to actively test your understanding and track your progress.
Stay Updated with the Latest in C++
The tech world evolves rapidly, and staying up-to-date is vital. This book incorporates the latest updates and best practices in C++, emphasizing adherence to coding standards like the C++ Core Guidelines. These guidelines stress the importance of static type safety, resource safety, and simplicity, enabling you to write clean and efficient code.
Visual Learning and Sample Programs
Visual representation plays a key role in understanding abstract concepts. This book integrates visual aids and sample programs to illustrate various language elements, enhancing your learning journey. Through engaging graphics and thoughtful design, you will find complex topics becoming more approachable.
Your Path to Mastery
Whether you are aiming to develop software applications, delve into game development, or enhance your career prospects, "Unlocking the Power of C++" is here to guide you every step of the way. Unlock your potential and dive into the dynamic world of C++ programming today!
Table of Contents
1. Getting Started with C++- Introduction to C++
- Installing a C++ Compiler
- Your First C++ Program: Hello World!
2. Understanding Basic Syntax
- Variables and Data Types
- Operators and Expressions
- Control Structures: If, Else, and Switch
3. Functions in C++
- Defining Functions
- Function Overloading
- The Importance of Scope
4. Object-Oriented Programming Basics
- Understanding Classes and Objects
- Encapsulation and Data Hiding
- Inheritance and Polymorphism
5. Advanced C++ Concepts
- Pointers and References
- Templates and Generic Programming
- Exception Handling
6. Working with the Standard Library
- Overview of the C++ Standard Library
- Using Containers: Vectors and Maps
- Working with Algorithms
7. Input and Output in C++
- Basic Input and Output Operations
- File I/O in C++
- String Streams
8. Practical Applications of C++
- Real-world Examples of C++ Usage
- Creating a Simple Application: Calculator Example
- Developing Games with C++
9. Testing and Debugging Techniques
- Importance of Testing Code
- Using Debuggers Effectively
- Writing Unit Tests in C++
10. C++ Best Practices
- Coding Standards and Guidelines
- Common Pitfalls and How to Avoid Them
- Maintaining Readable Code
11. Quizzes and Exercises
- Basic Syntax Quizzes
- Advanced Concepts Challenges
- Final Review Quiz
12. Conclusion and Next Steps
- Where to Go from Here
- Resources for Further Learning
- Community and Support in C++ Programming
Target Audience
This book is aimed at aspiring programmers, students, and professionals who are looking to deepen their understanding of C++ with practical examples and interactive learning methods.
Key Takeaways
- Deep understanding of C++ syntax and concepts.
- Ability to apply C++ in real-world programming scenarios.
- Skills to write clean, efficient code following best practices.
- Confidence in testing and debugging C++ applications.
- Engagement through quizzes and practical exercises to reinforce learning.
How This Book Was Generated
This book is the result of our advanced AI text generator, meticulously crafted to deliver not just information but meaningful insights. By leveraging our AI book generator, cutting-edge models, and real-time research, we ensure each page reflects the most current and reliable knowledge. Our AI processes vast data with unmatched precision, producing over 200 pages of coherent, authoritative content. This isn’t just a collection of facts—it’s a thoughtfully crafted narrative, shaped by our technology, that engages the mind and resonates with the reader, offering a deep, trustworthy exploration of the subject.
Satisfaction Guaranteed: Try It Risk-Free
We invite you to try it out for yourself, backed by our no-questions-asked money-back guarantee. If you're not completely satisfied, we'll refund your purchase—no strings attached.